Hey, developers welcome to Day 30 of our 90Days 90Projects challenge. And in Day 30 we are going to create a Toggle Dark Mode using HTML CSS and JavaScript
So to run this code you just need to copy the HTML and CSS code and run it into your code Editor.
Preview
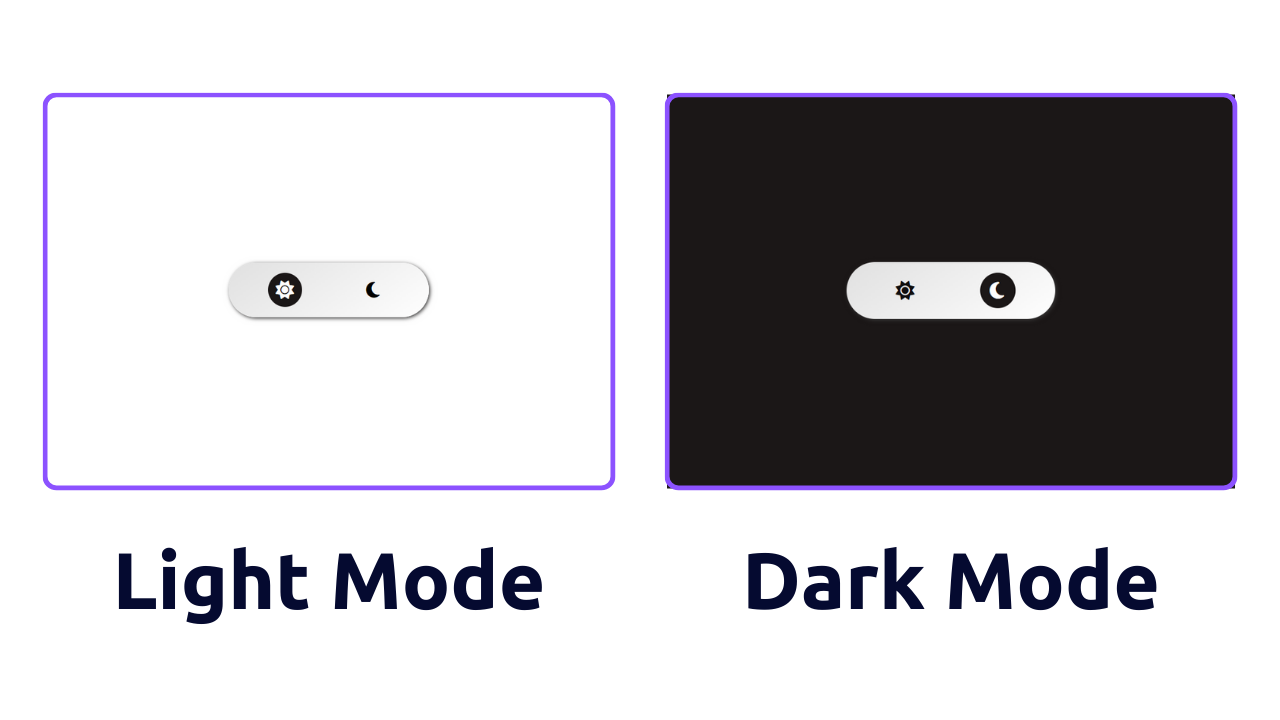
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title> Toggle Button</title>
<!-- linking stylesheets for toggle page -->
<link rel="stylesheet" href="style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.1/css/all.min.css"
integrity="sha512-MV7K8+y+gLIBoVD59lQIYicR65iaqukzvf/nwasF0nqhPay5w/9lJmVM2hMDcnK1OnMGCdVK+iQrJ7lzPJQd1w=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<label for="toggleBtn" class="toggleBtn">
<i class="fa-solid fa-sun"></i>
<i class="fa-solid fa-moon"></i>
<div class="bgMove"></div>
</label>
</body>
<!-- Adding Script -->
<script src="script.js"></script>
</html>
CSS Code
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
width: 100%;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background-color: white;
transition: all 0.4s linear;
}
body.dark {
background-color: rgb(27, 23, 23);
}
.toggleBtn {
z-index: 2;
padding: 1rem;
text-align: center;
border-radius: 5rem;
display: flex;
justify-content: space-between;
box-shadow: 1.5px 1.5px 5px #373636;
background: linear-gradient(145deg, #e1e1e1, #fff);
}
.bgMove {
width: 45px;
height: 45px;
position: absolute;
top: 50%;
z-index: -1;
border-radius: 50%;
transition: all 0.4s linear;
transform: translate(80%, -50%);
background-color: rgb(27, 23, 23);
}
.fa-sun {
color: white;
}
.bgMove.active {
color: white;
z-index: -2;
transition: all 0.4s linear;
transform: translate(340%, -50%);
background-color: rgb(27, 23, 23);
}
.dark {
color: rgb(16, 14, 14);
transition: all 0.4s linear;
}
.active {
color: white;
transition: all 0.4s linear;
}
.active body {
background-color: purple;
}
i {
z-index: 4;
width: 100px;
margin: .5rem;
cursor: pointer;
font-size: 1.5rem;
display: flex;
position: relative;
flex-direction: row;
justify-content: space-between;
transition: all 0.4s linear;
}
JavaScript
const btn = document.querySelector('.toggleBtn')
const moveBtn = document.querySelector('.bgMove')
const fa = document.querySelector('.fa-solid')
const faMoon = document.querySelector('.fa-moon')
btn.addEventListener('click', function () {
moveBtn.classList.toggle('active')
fa.classList.toggle('dark')
faMoon.classList.toggle('active')
if (moveBtn.classList == 'active') {
faMoon.classList.add('moon-active')
}
else {
faMoon.classList.remove('moon-active')
}
// Apply condition when toggle button is active and deactive
if (moveBtn.classList.contains('active')) {
document.body.classList.add('dark')
}
else {
document.body.classList.remove('dark')
}
})